Note: This is a guest post written by Tom Carrington – Unreal Engine is a powerful tool for game development, but like any other software, it requires optimization to achieve the best performance. In this article, we’ll explore some tips and tricks for optimizing performance in Unreal Engine game development. From tweaking settings to utilizing built-in tools, we’ll cover everything you need to know to get the most out of your Unreal Engine projects.
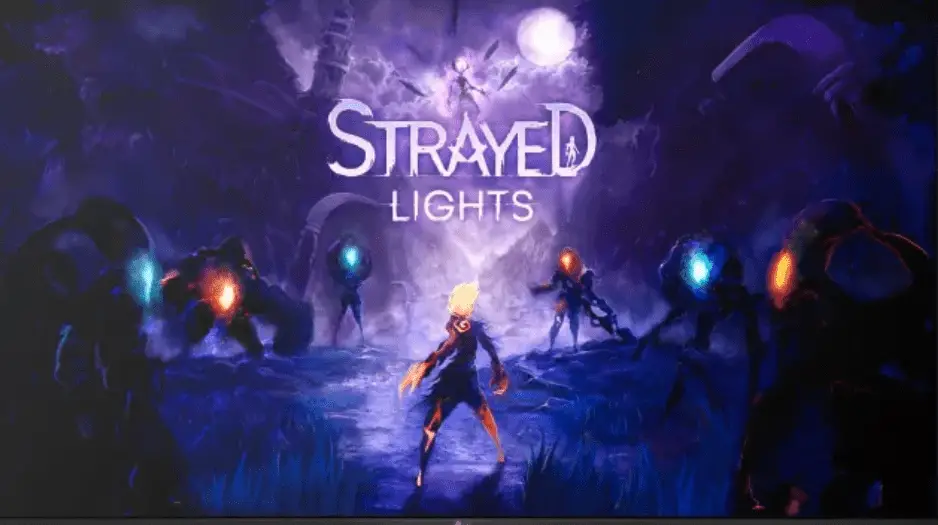
Section 1: Understanding Performance Metrics
In order to optimize performance in Unreal Engine, it’s important to first understand the key performance metrics that impact the overall performance of the game or application. Here are some important performance metrics and how to monitor and interpret them:
- Frame Rate: The frame rate is the number of frames per second that the game is rendering. It’s an important metric because a low frame rate can lead to stuttering and lag, making the game or application feel unresponsive. To monitor the frame rate, you can use Unreal Engine’s built-in console command “stat fps”. This will display the frame rate in the upper-right corner of the screen. A good target frame rate for most games is 60 frames per second.
- GPU Usage: The GPU (Graphics Processing Unit) is responsible for rendering the game’s graphics. Monitoring GPU usage is important because if the GPU is overloaded, it can cause the frame rate to drop. To monitor GPU usage, you can use the console command “stat gpu”. This will display the percentage of time the GPU is being used to render the game. If the GPU usage is consistently above 90%, you may need to optimize your graphics settings or reduce the complexity of your assets.
- CPU Usage: The CPU (Central Processing Unit) is responsible for running the game’s logic and AI. Monitoring CPU usage is important because if the CPU is overloaded, it can cause the game to slow down or even freeze. To monitor CPU usage, you can use the console command “stat unit”. This will display the percentage of time the CPU is being used for different tasks, such as game logic and physics calculations. If the CPU usage is consistently above 90%, you may need to optimize your code or reduce the complexity of your Blueprints.
- Memory Usage: Memory usage is the amount of RAM (Random Access Memory) being used by the game. Monitoring memory usage is important because if the game runs out of memory, it can cause crashes or performance issues. To monitor memory usage, you can use the console command “stat memory”. This will display the amount of memory being used by different parts of the game, such as textures and meshes. If the memory usage is consistently high, you may need to optimize your assets or reduce the number of assets in the scene.
By monitoring these key performance metrics and interpreting the data they provide, you can identify areas that need optimization and make adjustments to improve the overall performance of your game or application.
Section 2: Optimization Techniques for Level Design
Level design plays a crucial role in the overall performance of a game or application. Poorly optimized levels can cause significant performance issues, such as low frame rates, long loading times, and crashes. Here are some tips for optimizing level design in Unreal Engine:
- Reduce Draw Calls: Draw calls are the number of times the graphics card is called upon to render an object. Each draw call takes up processing time and memory, so reducing the number of draw calls is an effective way to improve performance. To reduce draw calls, you can merge multiple objects into a single mesh, use instanced meshes, or use the Hierarchical Instanced Static Mesh (HISM) feature in Unreal Engine.
- Use LODs: LODs (Level of Detail) are lower-detail versions of an asset that are used when the asset is far away from the camera. Using LODs can significantly reduce the number of polygons being rendered, which can improve performance. To use LODs in Unreal Engine, you can create multiple versions of an asset with decreasing levels of detail and assign them to the asset in the LOD settings.
- Optimize Lighting: Lighting can have a significant impact on performance, especially in dynamic lighting scenarios. To optimize lighting, you can use lightmaps, which pre-calculate lighting information for static objects and can significantly reduce the amount of lighting calculations that need to be done in real-time. You can also adjust the number of shadow-casting lights in the scene and adjust their shadow quality settings to balance visual quality with performance.
- Minimize Overdraw: Overdraw occurs when multiple layers of transparent objects are drawn on top of each other, causing the graphics card to render the same pixels multiple times. This can have a significant impact on performance, especially on lower-end hardware. To minimize overdraw, you can use materials with alpha testing instead of alpha blending, which eliminates the need for multiple layers of transparent objects.
Section 3: Optimization Techniques for Materials
Poorly optimized materials can cause performance issues, such as long loading times and low frame rates. Here are some tips for optimizing materials in Unreal Engine:
- Use Texture Atlases: Texture atlases are a collection of textures that have been combined into a single image. Using texture atlases can significantly reduce the number of texture samples being used, which can improve performance. To use texture atlases in Unreal Engine, you can create a single material that references the texture atlas and use texture coordinates to map the appropriate section of the atlas to each object.
- Reduce Shader Complexity: Shaders are the programs that run on the graphics card to render materials. Complex shaders can cause significant performance issues, especially on lower-end hardware. To reduce shader complexity, you can simplify the math operations used in the shader and remove any unnecessary calculations. You can also use the Shader Complexity view mode in Unreal Engine to identify areas of the scene that are using complex shaders.
- Use Material Instances: Material instances are copies of a master material that can be adjusted at runtime. Using material instances can significantly reduce the number of materials being used in a scene, which can improve performance. To use material instances in Unreal Engine, you can create a master material with all the desired parameters and then create instances that reference the master material.
- Use Static Lighting: Dynamic lighting can be very resource-intensive, especially on lower-end hardware. Using static lighting can significantly reduce the amount of lighting calculations that need to be done in real-time, which can improve performance. To use static lighting in Unreal Engine, you can use lightmass to pre-calculate lighting information for static objects.
Section 4: Optimization Techniques for Blueprints
Blueprints are a visual scripting system in Unreal Engine that allow developers to create gameplay logic and interactions without writing code. While Blueprints are powerful and easy to use, they can also have a significant impact on performance if not optimized properly. Here are some tips for optimizing Blueprints in Unreal Engine:
- Reduce Unnecessary Updates: Blueprints that update frequently can cause significant performance issues, especially if they are attached to many objects in the scene. To reduce unnecessary updates, you can use the “Tick Group” feature in Unreal Engine to specify when Blueprints should update. For example, if a Blueprint only needs to update once per second, you can set it to update on the “Second” tick group instead of the default “Every Frame” tick group.
- Use C++ for Heavy Computations: While Blueprints are great for prototyping and quick iterations, heavy computations such as complex math operations or physics simulations can cause significant performance issues. In these cases, it is often better to use C++ to write the logic directly. You can create a C++ class that inherits from the appropriate Blueprint class and then use that class in your game or application.
- Minimize Event Dispatchers: Event Dispatchers are a powerful feature in Blueprints that allow communication between different objects in the scene. However, overusing Event Dispatchers can cause significant performance issues. To minimize Event Dispatchers, you can use interfaces instead, which provide a similar functionality but are more lightweight.
- Use Replication Wisely: Replication is the process of synchronizing data between different clients in a multiplayer game. While replication is essential for multiplayer games, overusing replication can cause significant performance issues, especially in large-scale games. To use replication wisely, you can limit the amount of data being replicated and only replicate what is necessary for gameplay.
By following these tips and optimizing Blueprints, you can significantly improve the performance of your game or application, resulting in a better user experience and smoother gameplay.
Section 5: Optimization Techniques for Assets
Assets, such as 3D models, textures, and sound files, are an essential part of any game or application. Here are some tips for optimizing assets in Unreal Engine:
- Use Compressed Textures: High-resolution textures can take up a significant amount of memory and slow down loading times. To optimize textures, you can use texture compression to reduce their size without sacrificing visual quality. Unreal Engine supports various texture compression formats, such as DXT and BC, that can significantly reduce texture memory usage.
- Reduce Polygon Counts: High-polygon models can cause significant performance issues, especially on lower-end hardware. To reduce polygon counts, you can use tools such as the Unreal Engine Mesh Reduction Tool or third-party tools like Simplygon. These tools can automatically reduce the number of polygons in a model while preserving its visual quality.
- Use LODs: Level of Detail (LOD) is a technique used to reduce the number of polygons rendered for distant objects. By using LODs, you can significantly improve performance without sacrificing visual quality. Unreal Engine supports automatic LOD generation, which can generate multiple levels of detail for a model based on distance.
- Optimize Sound Files: Sound files can also have a significant impact on performance, especially if they are large or played frequently. To optimize sound files, you can reduce their bit rate, use compression, or cut unnecessary parts of the sound file. Unreal Engine supports various sound compression formats, such as OGG and MP3, that can significantly reduce file size without sacrificing audio quality.
Section 6: Optimization Techniques for Code
Code is a critical component of any game or application, and poorly optimized code can have a significant impact on performance. Here are some tips for optimizing code in Unreal Engine:
- Reduce Function Calls: Function calls can be expensive, especially if they are called frequently. To optimize code, you can reduce the number of function calls by consolidating code and reusing functions wherever possible. Additionally, you can use inline functions to reduce function call overhead.
- Avoid Dynamic Memory Allocation: Dynamic memory allocation, such as using “new” and “delete” operators, can be expensive and can cause memory fragmentation. To optimize code, you can use object pools and reuse objects instead of dynamically allocating and deallocating them. Additionally, you can use stack memory instead of heap memory for small objects to improve performance.
- Optimize Loops: Loops can be expensive, especially if they iterate over large arrays or collections. To optimize loops, you can use iterators or ranged-based loops to reduce the number of iterations. Additionally, you can use parallel algorithms or multi-threading to process data in parallel.
- Use Data Structures Wisely: Choosing the right data structure can have a significant impact on performance. For example, using a hash table can be faster than using a linear search. Additionally, you can use custom data structures, such as quad trees or octrees, to optimize collision detection or spatial queries.
Section 7: Optimization Techniques for Packaging and Distribution
A large package size can lead to long loading times and slow performance, especially on lower-end hardware. Here are some tips for optimizing packaging and distribution in Unreal Engine:
- Reduce Package Size: One of the best ways to optimize packaging and distribution is to reduce the package size. This can be achieved by removing unnecessary files and assets, such as debug symbols and unused assets. Additionally, you can use Unreal Engine’s asset manager to load assets on demand, reducing the size of the initial package.
- Use Compression: Compression can significantly reduce package size and improve loading times. Unreal Engine supports various compression formats, such as ZIP and Oodle, that can be used to compress packages and reduce their size.
- Optimize Streaming: Streaming can be used to load assets on demand and reduce the size of the initial package. To optimize streaming, you can use the asset manager to load assets dynamically based on the player’s location or progress in the game. Additionally, you can use level streaming to load only the necessary parts of the level, reducing loading times and memory usage.
- Use Delta Updates: Delta updates can be used to reduce the size of updates and patches by only downloading the changes between versions. Unreal Engine supports delta updates, which can significantly reduce the size of updates and improve download times.
The Bottom Line
In conclusion, optimizing performance in Unreal Engine is an ongoing process that is crucial for the success of any Unreal Engine project. By following the tips and tricks outlined in this article, you can ensure that your game runs smoothly and efficiently. Remember to continually monitor and optimize performance throughout the development process to achieve the best results. With a little effort and attention to detail, you can create a truly outstanding Unreal Engine game.